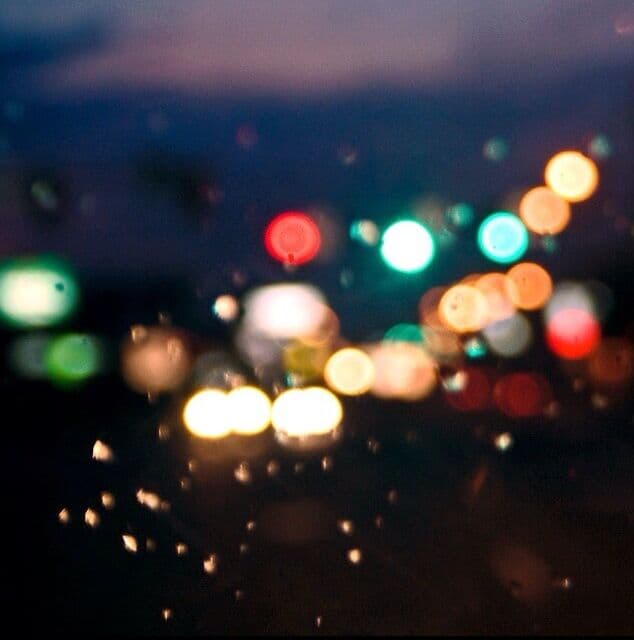
1、Iterator
一种数据结构只要部署了 Iterator 接口,我们就称这种数据结构是“可遍历的”(iterable)。
原生具备 Iterator 接口的数据结构如下:(==for…of可以遍历具备iterator的结构==)
-Array
-Map
-Set
-String
-TypedArray
-函数的 arguments 对象
-NodeList 对象
类似数组的对象调用数组的Symbol.iterator
方法
1 | let iterable = { |
1.1、调用Iterator的场合
数组的解构赋值
扩展运算符
yield* (后面跟的是一个可遍历的结构,它会调用该结构的遍历器接口)
其他场合
for…of
Array.form()
Map()、Set()
Promise.all()
Promise.race()
1.2、Array.form()方法用于将两类对象转为真正的数组:
类似数组的对象;
Iterator对象
2、Generator函数
2.1、yield表达式
由于 Generator 函数返回的遍历器对象,只有调用next
方法才会遍历下一个内部状态,所以其实提供了一种可以暂停执行的函数。yield
表达式就是暂停标志。
遍历器对象的next
方法的运行逻辑如下。
(1)==遇到yield
表达式,就暂停执行后面的操作==,并将紧跟在yield
==后面的那个表达式的值==,作为返回的对象的value
属性值。
(2)下一次调用next
方法时,再继续往下执行,直到遇到下一个yield
表达式。
(3)如果没有再遇到新的yield
表达式,就一直运行到函数结束,直到return
语句为止,并将return
语句后面的表达式的值,作为返回的对象的value
属性值。
(4)如果该函数没有return
语句,则返回的对象的value
属性值为undefined
。
1 | function* helloWorldGenerator() { |
2.2、与 Iterator 接口的关系
1 | var myIterable = {}; |
2.3、next参数
yield
表达式本身没有返回值,或者说总是返回undefined
。
next
方法可以带一个参数,该==参数就会被当作上一个yield
表达式的返回值==。
1 | function* foo(x) { |
2.4、for…of
1 | function* foo() { |
这里需要注意,一旦next
方法的返回对象的done
属性为true
,for...of
循环就会中止,且不包含该返回对象,所以上面代码的return
语句返回的6
,不包括在for...of
循环之中。
- Post title: ES6-Iterator
- Create time: 2020-10-12 13:15:00
- Post link: 2020/10/12/Iterator/
- Copyright notice: All articles in this blog are licensed under BY-NC-SA unless stating additionally.