JavaScript封装XMLHttpRequest请求
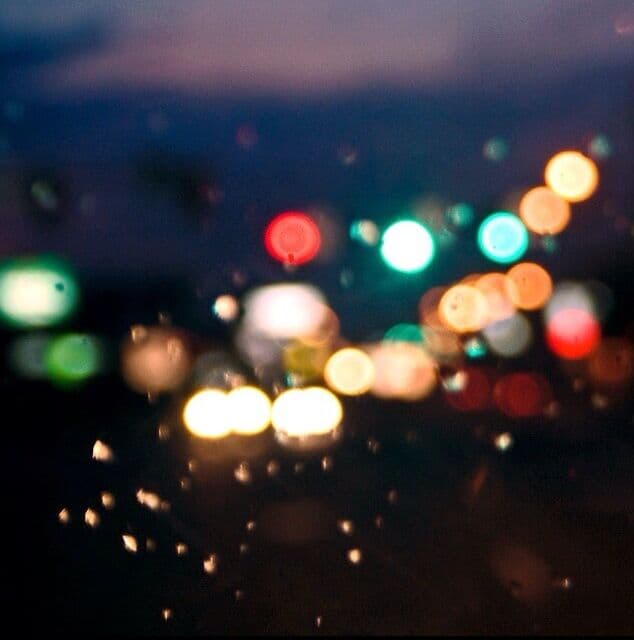
什么是 XMLHttpRequest 对象
XMLHttpRequest 对象用于在后台与服务器交换数据。
XMLHttpRequest 对象是开发者的梦想,因为您能够:
- 在不重新加载页面的情况下更新网页
- 在页面已加载后从服务器请求数据
- 在页面已加载后从服务器接收数据
- 在后台向服务器发送数据
所有现代的浏览器都支持 XMLHttpRequest 对象,XMLHttpRequest在 Ajax 编程中被大量使用。
原生javascript(ES5)封装XMLHttpRequest对象
1.创建ajax.js
1 | function Ajax(){} |
2.引用ajax.js
1 |
|
3.顺带看下后端写法(哈哈,虽然不需要前端开发人员操心)
4.最后看下四种请求的结果
ES6 封装XMLHttpRequest对象
同样ajax.js 文件
1 | const ajax = function(obj){ |
引用ajax.js
1 | <script type="module"> |
- Post title: JavaScript封装XMLHttpRequest请求
- Create time: 2018-08-16 13:48:00
- Post link: 2018/08/16/JavaScript封装XMLHttpRequest请求/
- Copyright notice: All articles in this blog are licensed under BY-NC-SA unless stating additionally.
Comments